Perfect Squares
Given a positive integer n, find the least number of perfect square numbers (for example, 1, 4, 9, 16, …) which sum to n.
(将一个正整数分为干个平方数的和,使得平方数个数最少)
Example:
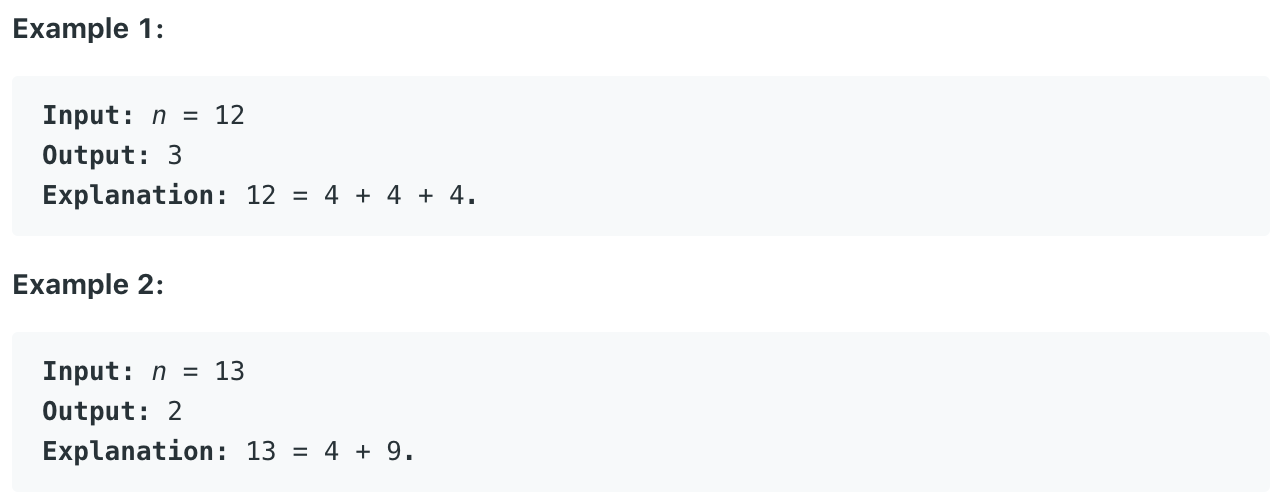
1. BFS
1 |
class : |
2. 动态规划
1 |
class : |
Given a positive integer n, find the least number of perfect square numbers (for example, 1, 4, 9, 16, …) which sum to n.
(将一个正整数分为干个平方数的和,使得平方数个数最少)
Example:
1 |
class : |
1 |
class : |
近期评论