Single Number II
Given a non-empty array of integers, every element appears three times except for one, which appears exactly once. Find that single one.
(只出现一次的数字(其余出现三次))
Note: Your algorithm should have a linear runtime complexity. Could you implement it without using extra memory?
Example:
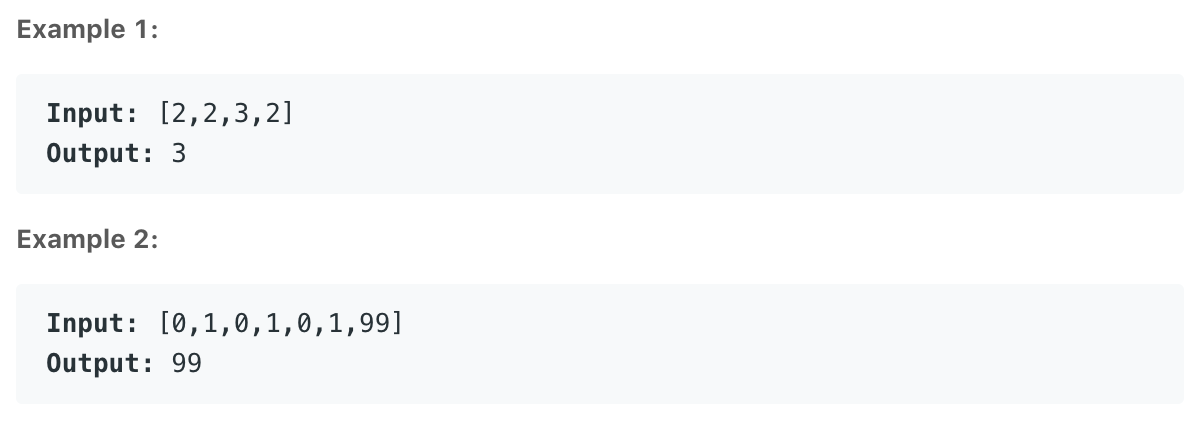
1. 按位操作
ones / twos / threes 表示将数组中所有整形数转为二进制后,每个位上1出现次数为1/2/3次的情况;
1 |
class : |
2. 使用Python库Counter
1 |
from collections import Counter |
近期评论