Distinct Subsequences
Given a string S and a string T, count the number of distinct subsequences of S which equals T. A subsequence of a string is a new string which is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, “ACE” is a subsequence of “ABCDE” while “AEC” is not).
(有效子序列个数)
Example:
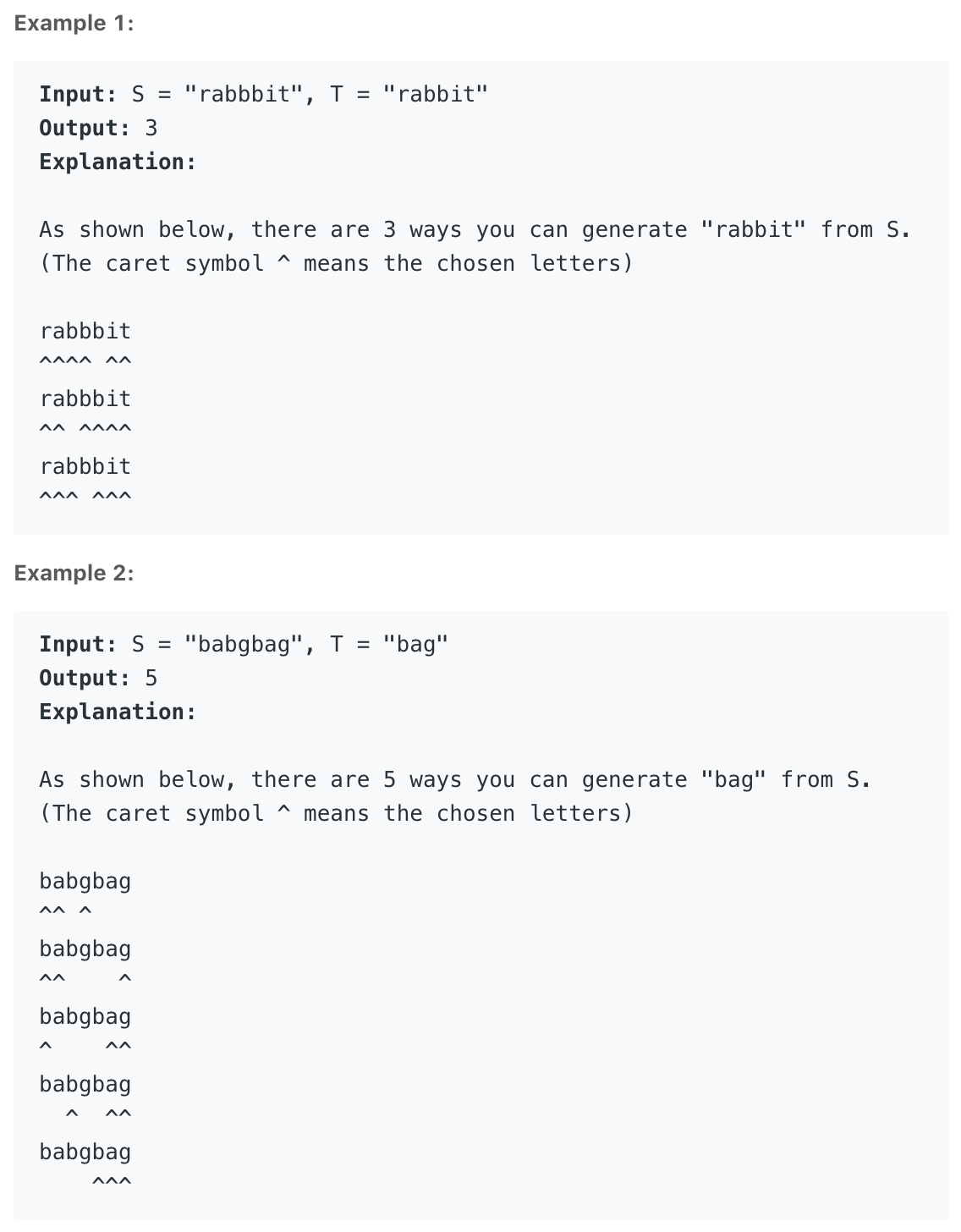
1. 动态规划
这个题也可以看做一个字符串匹配的问题,一般都可以使用动态规划求解。具体实现过程如下:
1 |
class : |
近期评论