一站式Java框架 核心 IoC(控制反转), AOP(面向切面编程) , 便于整合第三方框架
Spring特点
Spring的体系结构
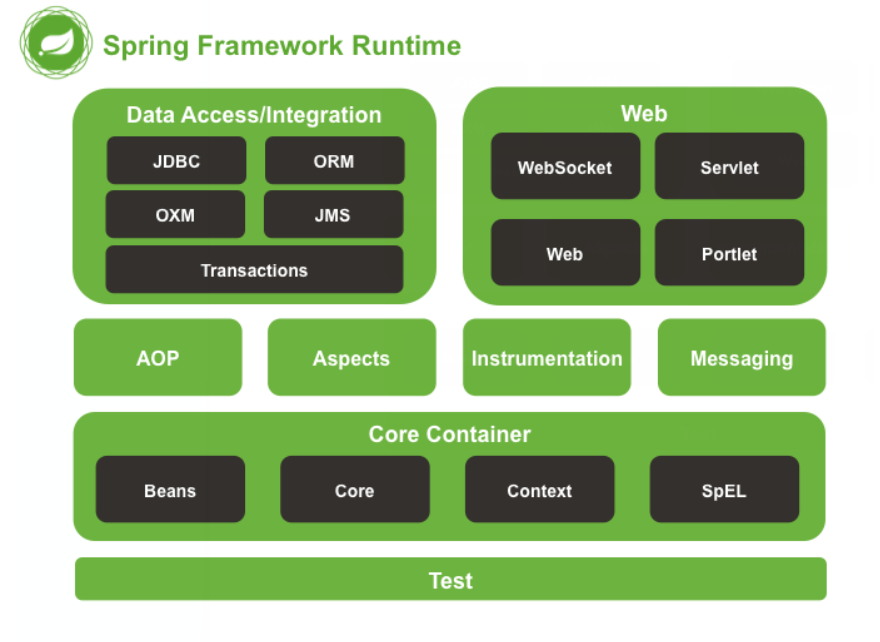
Core Contraniner : IoC
IoC 基础上提供了AOP
Spring 是容器, 底层是工厂 我们通过Spring来创建对象
结合JDBC分析程序中出现的问题
- 新建一手Maven项目
- pom.xml 引入坐标
- 补全目录 java, resources
- 写jdbc的Demo
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
public static void (String[] args) throws Exception{
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/eesy","root","1234"); PreparedStatement pstm = conn.prepareStatement("select * from account"); ResultSet rs = pstm.executeQuery(); while(rs.next()){ System.out.println(rs.getString("name")); } rs.close(); pstm.close(); conn.close(); }
|
- 发现一个问题 如果没有mysql的驱动 该程序就不能运行 这里就是 程序间的耦合
现在 解决类之间的依赖关系 , 降低类之间的依赖关系, 也就是 解耦
实际开发中做到 编译期不依赖, 运行时才依赖.
解耦的思路 :
- 使用反射来创建对象, 避免使用new
- 通过读取配置文件来获取创建对象的全限定类名
编写工厂类和配置文件完成解耦
Bean: 可重用组件 创建service和dao对象
JavaBean: Java编写的可重用组件
实体类: 通常与数据库有直接联系
- 搭建Java项目 标准三层架构
- 写
bean.properties
将 dao , service 以 key = value 形式 编写
- 编写
BeanFactory
读取 bean.properties
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
|
public class BeanFactory { private static Properties props;
private static Map<String,Object> beans;
static { try { props = new Properties(); InputStream in = BeanFactory.class.getClassLoader().getResourceAsStream("bean.properties"); props.load(in); beans = new HashMap<String,Object>(); Enumeration keys = props.keys(); while (keys.hasMoreElements()){ String key = keys.nextElement().toString(); String beanPath = props.getProperty(key); Object value = Class.forName(beanPath).newInstance(); beans.put(key,value); } }catch(Exception e){ throw new ExceptionInInitializerError("初始化properties失败!"); } }
public static Object getBean(String beanName){ return beans.get(beanName); }
}
|
近期评论